JSON is the acronym for JavaScript Object Notation, and it’s a format to exchange (and interchange) data from the same or different systems in a network.
JSON is a text format that is entirely language-independent but uses conventions similar to the C-family of languages. JSON is easy for humans to read and write, and it is easy for machines to parse and generate. These properties make JSON an ideal data-interchange language.

Two structures comprised JSON:
- A collection of name/value pairs.
- An ordered list of values.
We know the first structure in various languages as an object, record, struct, dictionary, hash table, keyed list, or associative array and the second structure array, vector, list, or sequence.
A JSON object is an unordered set of name/value pairs. An object begins with { and ends with }. Each name is followed by : (colon) and the name/value pairs are separated by, (comma).
{
"first name": "john",
"salary": 4000,
"married": true
}
A value in JSON could be an array. An array is an ordered collection of values. An array begins with [ and ends with ]. Values are separated by, (comma):
{
"First Name": "Alex",
"Job": "Teacher",
"Favorite cities": ["San Francisco", "California", "Houston"]
}
A value can be a string in double quotes, or a number, or true or false or null, or an object or an array. These structures can be nested.
{
"Grade": 3,
"Names":
[
{
"First name": "Jack",
"Last name": "London"
},
{
"First name": "Sam",
"Last name": "Neil"
},
{
"First name": "Peter",
"Last name": "Sellers"
}
]
}
JSON DataTypes
Any value in a JSON object must have one of the following datatypes.
Strings:
{“name”: “Jonathan”}
Number:
{“age”: 5}
Boolean:
{“married”: true}
Object:
{
“employee”: {“name”: “John”, “age”: 22}
}
The objects in the JSON objects that are nested must also follow the same syntax rules as that of a standard JSON object.
Serialization and DeSerialization
Serialization is the process of converting an object to a JSON object.
DeSerialization is the process of converting a JSON object to a programming language object.
There are a few methods in C# to accomplish Serialization and DeSerialization. In this article, I explain the Newtonsoft Json.NET (aka Newtonsoft.Json) method.
Json.NET is a library of methods for conversion between JSON object and .NET object using the JsonSerializer. The JsonSerializer converts .NET objects into their JSON equivalent by mapping the .NET object property names to the JSON property names.
You can add this library to your C# project from Nuget
Serialization
In Serialization, we convert a custom .Net object to a JSON string. In the following code, it creates an instance of Product and assigns some values to its properties. Then it calls static method SerializeObject() of JsonConvert class by passing object(Product). It returns JSON data in string format:
Product product = new Product();
product.Name = "Apple";
product.ExpiryDate = new DateTime(2008, 12, 28);
product.Price = 3.99M;
product.Sizes = new string[] { "Small", "Medium", "Large" };
string output = JsonConvert.SerializeObject(product);
The result JSON object (string):
{
"Name": "Apple",
"ExpiryDate": "2008-12-28T00:00:00",
"Price": 3.99,
"Sizes": [ "Small","Medium","Large" ]
}
Deserialization
In Deserialization, we convert a JSON object (string) to a custom .Net object. In the code below, I call the static method DeserializeObject() of the JsonConvert class by passing JSON data. It returns a custom object type of (Product) from JSON data.
string json=@"{
"Name": "Apple",
"ExpiryDate": "2008-12-28T00:00:00",
"Price": 3.99,
"Sizes": [ "Small","Medium","Large" ]
};
Product prodObj = JsonConvert.DeserializeObject<Product>(json);
Response.Write(prodObj.Name);
Other methods
DataContractJsonSerializer and JavaScriptJsonSerializer are the other two methods to work with JSON objects in a .Net environment.
However, Json.NET is faster, and it’s available as an open-source class.
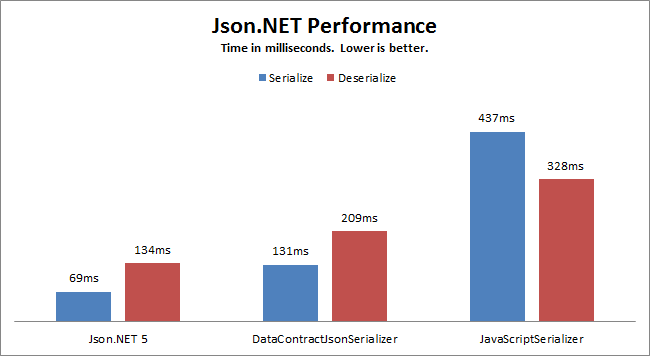
Exchange of objects
All the modern programming languages are capable of generating and handling dictionaries or hashes or associative arrays.
JSON is one the most common, if not the most common, data format in data exchange between the clients and the servers. Almost all modern APIs use JSON to exchange data with applications.
The following is a sample of a processed transaction and its JSON result from Clearent API:
{
"authorization-code": "AC789U",
"billing": {
"city": "St Louis",
"company": "Test Company",
"country": "United States",
"first-name": "John",
"last-name": "Doe",
"phone": "3148889999",
"state": "MO",
"street": "123 Example Street",
"street2": "Suite 700",
"zip": "85284"
},
"billing-is-shipping": "false",
"card": "4111111111111111",
"check-field-mid": true,
"client-ip": "127.0.0.1",
"comments": "Bad tipper .90 on 80.00 transaction",
"contactless": "false",
"contactless-device": "false",
"csc": "999",
"customer-id": "Customer 1234",
"description": "Transaction desc",
"email-address": "test@test.com",
"email-receipt": "false",
"encrypted-track-data": "string",
"invoice": "invoice123",
"is-amex": true,
"is-sp30": true,
"order-id": "99988d",
"pin-data": "string",
"platform-fees": [
{
"fee-name": "DealManager"
}
],
"purchase-order": "PO789",
"sales-tax-amount": "90.00",
"sales-tax-type": "LOCAL_SALES_TAX",
"service-fee": "2.90",
"shipping": {
"city": "St Louis",
"company": "Test Company",
"country": "United States",
"first-name": "John",
"last-name": "Doe",
"phone": "3148889999",
"state": "MO",
"street": "123 Example Street",
"street2": "Suite 700",
"zip": "85284"
},
"software-type": "MySoftware_Verrsion1.3",
"tip-amount": "0.90",
"track2-data": "string",
"type": "SALE",
"amount": "80.00",
"exp-date": "0932",
"id": "string",
"software-type-version": "string",
"card-type": "VISA",
"create-token": "false",
"merchant-legacy-token": "false",
"token-description": "string",
"track-format": "MAGTEK",
"check-field": "invoice",
"cvm": "NON (None)",
"emv-data": "string",
"emv-entry-method": "EMV_DIP",
"key-serial-number": "string"
}
Downsides
We saw the benefits of JSON, but it is not devoid of downsides and disadvantages too. Following are the disadvantages:
- It is not fully secure.
- It is limited in terms of the supported data types (Boolean, String, Number, and object).
JSON is a great data format for accessing, storing, and exchanging data. If you are looking for something quick, lightweight, and simple for data exchange, JSON is your best option.